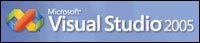
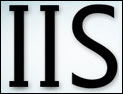
Failed to access IIS metabase.
The process account used to run ASP.NET must have read access to the IIS metabase (e.g. IIS://servername/W3SVC).
I was referred to a Microsoft Knowledge Base article. The problem supposedly happened because the ASP.NET worker process didn't have permissions to the mystery that is the IIS metabase. My guess is that this ocurred because I installed IIS after I installed Visual Studio. Microsoft recommended running the following command line as a remedy:
aspnet_regiis –ga [WindowsUserAccount]
I substituted ASPNET (the ASP.NET account on Windows XP) in for [WindowsUserAccount]. Unfortunately this did not solve the problem and I continued getting the same error mentioned above.
After searching some forums I found others who had encountered this issue who advised reinstalling the .NET 2.0 Framework. I went ahead and did this as follows and it resolved the incident:
- Run the Visual Studio 2005 Disk
- Select "Change or Remove Visual Studio 2005"
- Choose Repair/Reinstall