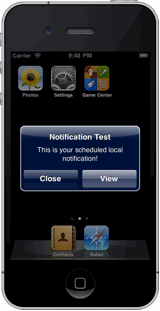
iOS 4 introduced local notifications, allowing apps to communicate brief text messages to users. In particular, a scheduled local notification can reach a user whether the app is running in the foreground, in the background or not running at all. While not as versatile as push notifications, scheduled local notifications can be helpful when an app needs to set-up predetermined alarms or reminders. Each app can have a total of 64 simultaneous scheduled local notifications (use them wisely so as to not annoy your users).
Below is an example of how to schedule a UILocalNotification using MonoTouch:
//Schedule one minute from the time of execution with no repeat
UILocalNotification notification = new UILocalNotification{
FireDate = DateTime.Now.AddMinutes(1),
TimeZone = NSTimeZone.LocalTimeZone,
AlertBody = "This is your scheduled local notification!",
RepeatInterval = 0
};
UIApplication.SharedApplication.ScheduleLocalNotification(notification);
You might notice that scheduled local notifications are automatically displayed when the current date/time surpasses our
FireDate and the app is in the background or not running at all. However, when the app is in the foreground local notifications are seemingly ignored. This is by design. If the app is in the foreground you are in charge of responding to scheduled local notifications by overriding the
ReceivedLocalNotification method as follows:
public override void ReceivedLocalNotification(UIApplication application,
UILocalNotification notification)
{
//Do something to respond to the scheduled local notification
UIAlertView alert = new UIAlertView("Notification Test",
notification.AlertBody, null, "Okay");
alert.Show();
}
1 comment:
Thank you very much for posting this!
Post a Comment